Entity Framework 6 with PostgreSQL
Pretty much like the last post with mysql I will use the concept of DataBase First but without using the Visual Studio wizard to create the Edmx file, so instead of that, I will do it manually again. I will create a Console Application again, so first install the next packages
Install-Package EntityFramework
Install-Package Npgsql
Install-Package Npgsql.EntityFramework
Be sure that you have already installed the npgsql provider or check this link
Add the connection string in the app.confing
<connectionStrings>
<add name="MonkeyFist" connectionString="server=localhost;user id=myuser;password=mypass;database=mydatabase" providerName="Npgsql" />
</connectionStrings>
the app.confing should be like this
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<configSections>
<!-- For more information on Entity Framework configuration, visit http://go.microsoft.com/fwlink/?LinkID=237468 -->
<section name="entityFramework" type="System.Data.Entity.Internal.ConfigFile.EntityFrameworkSection, EntityFramework, Version=6.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" requirePermission="false" />
</configSections>
<connectionStrings>
<add name="MonkeyFist" connectionString="server=localhost;user id=myuser;password=mypass;database=mydatabase" providerName="Npgsql" />
</connectionStrings>
<entityFramework>
<defaultConnectionFactory type="System.Data.Entity.Infrastructure.SqlConnectionFactory, EntityFramework" />
<providers>
<provider invariantName="Npgsql" type="Npgsql.NpgsqlServices, Npgsql.EntityFramework" />
</providers>
</entityFramework>
</configuration>
the Entity
[Table("customer", Schema = "public")]
public class Customer
{
[Key]
[Column("id_customer")]
public int id { get; set; }
public string customer { get; set; }
public string nit { get; set; }
public string address { get; set; }
}
the Context
public partial class db_Entities : DbContext
{
public db_Entities() : base(nameOrConnectionString: "MonkeyFist") { }
public DbSet<Customer> Customer { get; set; }
}
class Program
{
static void Main(string[] args)
{
using (var context = new db_Entities())
{
var customers = context.Customer.ToList();
foreach (var cust in customers)
{
Console.WriteLine(cust.id + " " + cust.customer + " " + cust.address);
}
}
}
}
the result
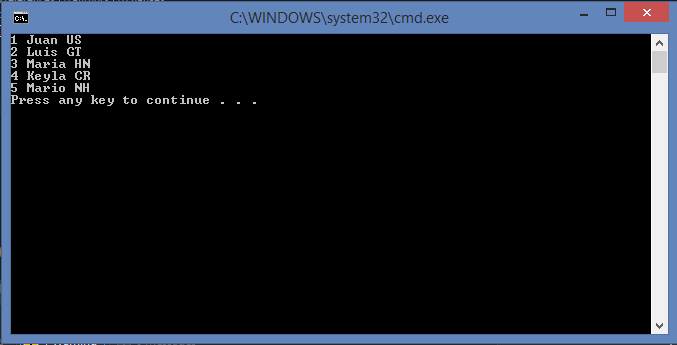